Struct definitions for core object types. More...
#include <stdio.h>
#include "kuroko.h"
#include "value.h"
#include "chunk.h"
#include "table.h"
#include <pthread.h>
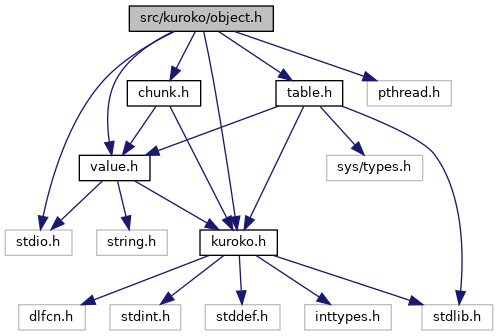

Go to the source code of this file.
Data Structures | |
struct | KrkObj |
The most basic object type. More... | |
struct | KrkString |
Immutable sequence of Unicode codepoints. More... | |
struct | KrkBytes |
Immutable sequence of bytes. More... | |
struct | KrkUpvalue |
Storage for values referenced from nested functions. More... | |
struct | KrkLocalEntry |
Metadata on a local variable name in a function. More... | |
struct | KrkExpressionsMap |
Map entry of opcode offsets to expressions spans. More... | |
struct | KrkOverlongJump |
struct | KrkCodeObject |
Code object. More... | |
struct | KrkClosure |
Function object. More... | |
struct | KrkClass |
Type object. More... | |
struct | KrkInstance |
An object of a class. More... | |
struct | KrkBoundMethod |
A function that has been attached to an object to serve as a method. More... | |
struct | KrkNative |
Managed binding to a C function. More... | |
struct | KrkTuple |
Immutable sequence of arbitrary values. More... | |
struct | KrkList |
Mutable array of values. More... | |
struct | KrkDict |
Flexible mapping type. More... | |
struct | DictItems |
struct | DictKeys |
struct | DictValues |
struct | KrkModule |
Representation of a loaded module. More... | |
struct | KrkSlice |
Macros | |
#define | KRK_OBJ_FLAGS_STRING_MASK 0x0003 |
#define | KRK_OBJ_FLAGS_STRING_ASCII 0x0000 |
#define | KRK_OBJ_FLAGS_STRING_UCS1 0x0001 |
#define | KRK_OBJ_FLAGS_STRING_UCS2 0x0002 |
#define | KRK_OBJ_FLAGS_STRING_UCS4 0x0003 |
#define | KRK_OBJ_FLAGS_CODEOBJECT_COLLECTS_ARGS 0x0001 |
#define | KRK_OBJ_FLAGS_CODEOBJECT_COLLECTS_KWS 0x0002 |
#define | KRK_OBJ_FLAGS_CODEOBJECT_IS_GENERATOR 0x0004 |
#define | KRK_OBJ_FLAGS_CODEOBJECT_IS_COROUTINE 0x0008 |
#define | KRK_OBJ_FLAGS_FUNCTION_MASK 0x0003 |
#define | KRK_OBJ_FLAGS_FUNCTION_IS_CLASS_METHOD 0x0001 |
#define | KRK_OBJ_FLAGS_FUNCTION_IS_STATIC_METHOD 0x0002 |
#define | KRK_OBJ_FLAGS_NO_INHERIT 0x0200 |
#define | KRK_OBJ_FLAGS_SECOND_CHANCE 0x0100 |
#define | KRK_OBJ_FLAGS_IS_MARKED 0x0010 |
#define | KRK_OBJ_FLAGS_IN_REPR 0x0020 |
#define | KRK_OBJ_FLAGS_IMMORTAL 0x0040 |
#define | KRK_OBJ_FLAGS_VALID_HASH 0x0080 |
#define | krk_isObjType(v, t) (IS_OBJECT(v) && (AS_OBJECT(v)->type == (t))) |
#define | OBJECT_TYPE(value) (AS_OBJECT(value)->type) |
#define | IS_STRING(value) krk_isObjType(value, KRK_OBJ_STRING) |
#define | AS_STRING(value) ((KrkString *)AS_OBJECT(value)) |
#define | AS_CSTRING(value) (((KrkString *)AS_OBJECT(value))->chars) |
#define | IS_BYTES(value) krk_isObjType(value, KRK_OBJ_BYTES) |
#define | AS_BYTES(value) ((KrkBytes*)AS_OBJECT(value)) |
#define | IS_NATIVE(value) krk_isObjType(value, KRK_OBJ_NATIVE) |
#define | AS_NATIVE(value) ((KrkNative *)AS_OBJECT(value)) |
#define | IS_CLOSURE(value) krk_isObjType(value, KRK_OBJ_CLOSURE) |
#define | AS_CLOSURE(value) ((KrkClosure *)AS_OBJECT(value)) |
#define | IS_CLASS(value) krk_isObjType(value, KRK_OBJ_CLASS) |
#define | AS_CLASS(value) ((KrkClass *)AS_OBJECT(value)) |
#define | IS_INSTANCE(value) krk_isObjType(value, KRK_OBJ_INSTANCE) |
#define | AS_INSTANCE(value) ((KrkInstance *)AS_OBJECT(value)) |
#define | IS_BOUND_METHOD(value) krk_isObjType(value, KRK_OBJ_BOUND_METHOD) |
#define | AS_BOUND_METHOD(value) ((KrkBoundMethod*)AS_OBJECT(value)) |
#define | IS_TUPLE(value) krk_isObjType(value, KRK_OBJ_TUPLE) |
#define | AS_TUPLE(value) ((KrkTuple *)AS_OBJECT(value)) |
#define | AS_LIST(value) (&((KrkList *)AS_OBJECT(value))->values) |
#define | AS_DICT(value) (&((KrkDict *)AS_OBJECT(value))->entries) |
#define | IS_codeobject(value) krk_isObjType(value, KRK_OBJ_CODEOBJECT) |
#define | AS_codeobject(value) ((KrkCodeObject *)AS_OBJECT(value)) |
Typedefs | |
typedef struct KrkObj | KrkObj |
The most basic object type. More... | |
typedef struct KrkString | KrkString |
Immutable sequence of Unicode codepoints. | |
typedef struct KrkUpvalue | KrkUpvalue |
Storage for values referenced from nested functions. | |
typedef void(* | KrkCleanupCallback) (struct KrkInstance *) |
typedef struct KrkClass | KrkClass |
Type object. More... | |
typedef struct KrkInstance | KrkInstance |
An object of a class. More... | |
typedef KrkValue(* | NativeFn) (int argCount, const KrkValue *args, int hasKwargs) |
Enumerations | |
enum | KrkObjType { KRK_OBJ_CODEOBJECT , KRK_OBJ_NATIVE , KRK_OBJ_CLOSURE , KRK_OBJ_STRING , KRK_OBJ_UPVALUE , KRK_OBJ_CLASS , KRK_OBJ_INSTANCE , KRK_OBJ_BOUND_METHOD , KRK_OBJ_TUPLE , KRK_OBJ_BYTES } |
Union tag for heap objects. More... | |
enum | KrkStringType { KRK_STRING_ASCII = KRK_OBJ_FLAGS_STRING_ASCII , KRK_STRING_UCS1 = KRK_OBJ_FLAGS_STRING_UCS1 , KRK_STRING_UCS2 = KRK_OBJ_FLAGS_STRING_UCS2 , KRK_STRING_UCS4 = KRK_OBJ_FLAGS_STRING_UCS4 } |
String compact storage type. More... | |
Functions | |
int | krk_getAwaitable (void) |
Calls await | |
Variables | |
NativeFn | krk_GenericAlias |
Special value for type hint expressions. More... | |
Detailed Description
Struct definitions for core object types.
Definition in file object.h.
Typedef Documentation
◆ KrkClass
Type object.
Represents classes defined in user code as well as classes defined by C extensions to represent method tables for new types.
◆ KrkInstance
typedef struct KrkInstance KrkInstance |
An object of a class.
Created by class initializers, instances are the standard type of objects built by managed code. Not all objects are instances, but all instances are objects, and all instances have well-defined class.
◆ KrkObj
The most basic object type.
This is the base of all object types and contains the core structures for garbage collection.
Enumeration Type Documentation
◆ KrkObjType
enum KrkObjType |
◆ KrkStringType
enum KrkStringType |
String compact storage type.
Indicates how raw codepoint values are stored in an a string object. We use the smallest form, of the four options, that can provide direct lookup by codepoint index for all codepoints. ASCII and UCS1 are separated because ASCII can re-use the existing UTF8 encoded data instead of allocating a separate buffer for codepoints, but this is not possible for UCS1.
Variable Documentation
◆ krk_GenericAlias
|
extern |
Special value for type hint expressions.
Returns a generic alias object. Bind this to a class's __class_getitem__ to allow for generic collection types to be used in type hints.
Definition at line 67 of file obj_typing.c.