Type object. More...
#include <object.h>
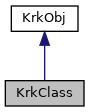
Public Member Functions | |
KrkClass * | krk_newClass (KrkString *name, KrkClass *base) |
Create a new class object. More... | |
int | krk_bindMethod (KrkClass *_class, KrkString *name) |
Perform method binding on the stack. More... | |
int | krk_bindMethodSuper (KrkClass *baseClass, KrkString *name, KrkClass *realClass) |
Bind a method with super() semantics. More... | |
KrkClass * | krk_makeClass (KrkInstance *module, KrkClass **_class, const char *name, KrkClass *base) |
Convenience function for creating new types. More... | |
void | krk_finalizeClass (KrkClass *_class) |
Finalize a class by collecting pointers to core methods. More... | |
Data Fields | |
struct KrkClass * | _class |
Metaclass. | |
KrkTable | methods |
General attributes table. | |
KrkString * | name |
Name of the class. | |
KrkString * | filename |
Filename of the original source that defined the codeobject for the class. | |
struct KrkClass * | base |
Pointer to base class implementation. | |
size_t | allocSize |
Size to allocate when creating instances of this class. | |
KrkCleanupCallback | _ongcscan |
C function to call when the garbage collector visits an instance of this class in the scan phase. | |
KrkCleanupCallback | _ongcsweep |
C function to call when the garbage collector is discarding an instance of this class. | |
KrkTable | subclasses |
Set of classes that subclass this class. | |
KrkObj * | _getter |
__getitem__ Called when an instance is subscripted | |
KrkObj * | _setter |
__setitem__ Called when a subscripted instance is assigned to | |
KrkObj * | _reprer |
__repr__ Called to create a reproducible string representation of an instance | |
KrkObj * | _tostr |
__str__ Called to produce a string from an instance | |
KrkObj * | _call |
__call__ Called when an instance is called like a function | |
KrkObj * | _init |
__init__ Implicitly called when an instance is created | |
KrkObj * | _eq |
__eq__ Implementation for equality check (==) | |
KrkObj * | _len |
__len__ Generally called by len() but may be used to determine truthiness | |
KrkObj * | _enter |
__enter__ Called upon entry into a with block | |
KrkObj * | _exit |
__exit__ Called upon exit from a with block | |
KrkObj * | _delitem |
__delitem__ Called when del is used with a subscript | |
KrkObj * | _iter |
__iter__ Called by for ... in ... , etc. | |
KrkObj * | _getattr |
__getattr__ Overrides normal behavior for attribute access | |
KrkObj * | _dir |
__dir__ Overrides normal behavior for dir() | |
KrkObj * | _contains |
__contains__ Called to resolve in (as a binary operator) | |
KrkObj * | _descget |
__get__ Called when a descriptor object is bound as a property | |
KrkObj * | _descset |
__set__ Called when a descriptor object is assigned to as a property | |
KrkObj * | _classgetitem |
__class_getitem__ Class method called when a type object is subscripted; used for type hints | |
KrkObj * | _hash |
__hash__ Called when an instance is a key in a dict or an entry in a set | |
KrkObj * | _add |
KrkObj * | _radd |
KrkObj * | _iadd |
KrkObj * | _sub |
KrkObj * | _rsub |
KrkObj * | _isub |
KrkObj * | _mul |
KrkObj * | _rmul |
KrkObj * | _imul |
KrkObj * | _or |
KrkObj * | _ror |
KrkObj * | _ior |
KrkObj * | _xor |
KrkObj * | _rxor |
KrkObj * | _ixor |
KrkObj * | _and |
KrkObj * | _rand |
KrkObj * | _iand |
KrkObj * | _mod |
KrkObj * | _rmod |
KrkObj * | _imod |
KrkObj * | _pow |
KrkObj * | _rpow |
KrkObj * | _ipow |
KrkObj * | _lshift |
KrkObj * | _rlshift |
KrkObj * | _ilshift |
KrkObj * | _rshift |
KrkObj * | _rrshift |
KrkObj * | _irshift |
KrkObj * | _truediv |
KrkObj * | _rtruediv |
KrkObj * | _itruediv |
KrkObj * | _floordiv |
KrkObj * | _rfloordiv |
KrkObj * | _ifloordiv |
KrkObj * | _lt |
KrkObj * | _gt |
KrkObj * | _le |
KrkObj * | _ge |
KrkObj * | _invert |
KrkObj * | _negate |
KrkObj * | _set_name |
KrkObj * | _matmul |
KrkObj * | _rmatmul |
KrkObj * | _imatmul |
KrkObj * | _pos |
KrkObj * | _setattr |
KrkObj * | _format |
KrkObj * | _new |
KrkObj * | _bool |
size_t | cacheIndex |
![]() | |
uint16_t | type |
Tag indicating core type. | |
uint16_t | flags |
General object flags, mostly related to garbage collection. | |
uint32_t | hash |
Cached hash value for table keys. | |
struct KrkObj * | next |
Invasive linked list of all objects in the VM. | |
Protected Attributes | |
KrkObj | obj |
Base. | |
Detailed Description
Type object.
Represents classes defined in user code as well as classes defined by C extensions to represent method tables for new types.
Member Function Documentation
◆ krk_bindMethod()
Perform method binding on the stack.
Performs attribute lookup from the class _class
for name
. If name
is not a valid member, the binding fails. If name
is a valid method, the method will be retrieved and bound to the instance on the top of the stack, replacing it with a BoundMethod object. If name
is not a method, the unbound attribute is returned. If name
is a descriptor, the __get__
method is executed.
- Parameters
-
_class Class object to resolve methods from. name String object with the name of the method to resolve.
- Returns
- 1 if the method has been bound, 0 if binding failed.
◆ krk_bindMethodSuper()
Bind a method with super() semantics.
- See also
- krk_bindMethod
Allows binding potential class methods with the correct class object while searching from a base class. Used by the super()
mechanism.
- Parameters
-
baseClass The superclass to begin searching from. name The name of the member to look up. realClass The class to bind if a class method is found.
- Returns
- 1 if a member has been found, 0 if binding fails.
◆ krk_finalizeClass()
void krk_finalizeClass | ( | KrkClass * | _class | ) |
Finalize a class by collecting pointers to core methods.
Scans through the methods table of a class object to find special methods and assign them to the class object's pointer table so they can be referenced directly without performing hash lookups.
- Parameters
-
_class Class object to finalize.
◆ krk_makeClass()
KrkClass * krk_makeClass | ( | KrkInstance * | module, |
KrkClass ** | _class, | ||
const char * | name, | ||
KrkClass * | base | ||
) |
Convenience function for creating new types.
Creates a class object, output to _class
, setting its name to name
, inheriting from base
, and attaching it with its name to the fields table of the given module
.
- Parameters
-
module Pointer to an instance for a module to attach to, or NULL
to skip attaching._class Output pointer to assign the new class object to. name Name of the new class. base Pointer to class object to inherit from.
- Returns
- A pointer to the class object, equivalent to the value assigned to
_class
.
◆ krk_newClass()
Create a new class object.
Creates a new class with the give name and base class. Generally, you will want to use krk_makeClass instead, which handles binding the class to a module.
The documentation for this struct was generated from the following files: