Functions for dealing with garbage collection and memory allocation. More...
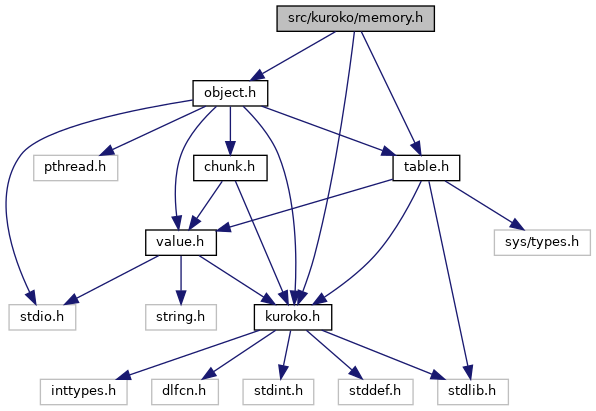

Go to the source code of this file.
Macros | |
#define | KRK_GROW_CAPACITY(c) ((c) < 8 ? 8 : (c) * 2) |
#define | KRK_GROW_ARRAY(t, p, o, n) (t*)krk_reallocate(p,sizeof(t)*o,sizeof(t)*n) |
#define | KRK_FREE_ARRAY(t, a, c) krk_reallocate(a,sizeof(t) * c, 0) |
#define | KRK_ALLOCATE(type, count) (type*)krk_reallocate(NULL,0,sizeof(type)*(count)) |
Functions | |
void * | krk_reallocate (void *ptr, size_t old, size_t new) |
Resize an allocated heap object. More... | |
void | krk_freeObjects (void) |
Release all objects. More... | |
size_t | krk_collectGarbage (void) |
Run a cycle of the garbage collector. More... | |
void | krk_markValue (KrkValue value) |
During a GC scan cycle, mark a value as used. More... | |
void | krk_markObject (KrkObj *object) |
During a GC scan cycle, mark an object as used. More... | |
void | krk_markTable (KrkTable *table) |
During a GC scan cycle, mark the contents of a table as used. More... | |
void | krk_gcTakeBytes (const void *ptr, size_t size) |
Assume ownership of size bytes at ptr . More... | |
Detailed Description
Functions for dealing with garbage collection and memory allocation.
Definition in file memory.h.
Function Documentation
◆ krk_collectGarbage()
size_t krk_collectGarbage | ( | void | ) |
Run a cycle of the garbage collector.
Runs one scan-sweep cycle of the garbage collector, potentially freeing unused resources and advancing potentially-unused resources to the next stage of removal.
- Returns
- The number of bytes released by this collection cycle.
The GC scheduling is in need of some improvement. The strategy at the moment is to schedule the next collect at double the current post-collection byte allocation size, up until that reaches 128MiB (64*2). Beyond that point, the next collection is scheduled for 64MiB after the current value.
Previously, we always doubled as that was what Lox did, but this rather quickly runs into issues when memory allocation climbs into the GiB range. 64MiB seems to be a good switchover point.
◆ krk_freeObjects()
void krk_freeObjects | ( | void | ) |
Release all objects.
Generally called automatically by krk_freeVM(); releases all of the GC-tracked heap objects.
◆ krk_gcTakeBytes()
void krk_gcTakeBytes | ( | const void * | ptr, |
size_t | size | ||
) |
Assume ownership of size
bytes at ptr
.
Future memory allocation operations with this ptr must be through krk_reallocate
- size
will be added to the VM allocation count, and if extensively memory debugging is enabled then the pointer will be marked as owned.
- Parameters
-
ptr Pointer to take ownership of size Size of data at ptr
◆ krk_markObject()
void krk_markObject | ( | KrkObj * | object | ) |
◆ krk_markTable()
void krk_markTable | ( | KrkTable * | table | ) |
◆ krk_markValue()
void krk_markValue | ( | KrkValue | value | ) |
◆ krk_reallocate()
void* krk_reallocate | ( | void * | ptr, |
size_t | old, | ||
size_t | new | ||
) |
Resize an allocated heap object.
Allocates or reallocates the heap object 'ptr', tracking changes in sizes from 'old' to 'new'. If 'ptr' is NULL, 'old' should be 0, and a new pointer will be allocated of size 'new'.
- Parameters
-
ptr Heap object to resize. old Current size of the object. new New size of the object.
- Returns
- New pointer for heap object.