Functions for debugging bytecode execution. More...
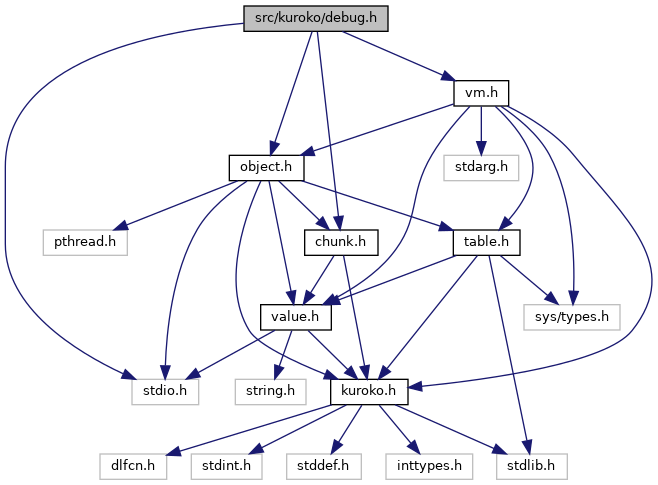

Go to the source code of this file.
Macros | |
#define | KRK_BREAKPOINT_NORMAL 0 |
#define | KRK_BREAKPOINT_ONCE 1 |
#define | KRK_BREAKPOINT_REPEAT 2 |
#define | KRK_DEBUGGER_CONTINUE 0 |
#define | KRK_DEBUGGER_ABORT 1 |
#define | KRK_DEBUGGER_STEP 2 |
#define | KRK_DEBUGGER_RAISE 3 |
#define | KRK_DEBUGGER_QUIT 4 |
Typedefs | |
typedef int(* | KrkDebugCallback) (KrkCallFrame *frame) |
Function pointer for a debugger hook. krk_debug_registerCallback() | |
Functions | |
void | krk_disassembleCodeObject (FILE *f, KrkCodeObject *func, const char *name) |
Print a disassembly of 'func' to the stream 'f'. More... | |
size_t | krk_disassembleInstruction (FILE *f, KrkCodeObject *func, size_t offset) |
Print a disassembly of a single opcode instruction. More... | |
int | krk_debugBreakpointHandler (void) |
Called by the VM when a breakpoint is encountered. More... | |
int | krk_debuggerHook (KrkCallFrame *frame) |
Called by the VM on single step. More... | |
int | krk_debug_registerCallback (KrkDebugCallback hook) |
Register a debugger callback. More... | |
int | krk_debug_addBreakpointFileLine (KrkString *filename, size_t line, int flags) |
Add a breakpoint to the given line of a file. More... | |
int | krk_debug_addBreakpointCodeOffset (KrkCodeObject *codeObject, size_t offset, int flags) |
Add a breakpoint to the given code object. More... | |
int | krk_debug_removeBreakpoint (int breakpointId) |
Remove a breakpoint from the breakpoint table. More... | |
int | krk_debug_enableBreakpoint (int breakpointId) |
Enable a breakpoint. More... | |
int | krk_debug_disableBreakpoint (int breakpointId) |
Disable a breakpoint. More... | |
void | krk_debug_enableSingleStep (void) |
Enable single stepping in the current thread. | |
void | krk_debug_disableSingleStep (void) |
Disable single stepping in the current thread. | |
void | krk_debug_dumpTraceback (void) |
Safely dump a traceback to stderr. More... | |
int | krk_debug_examineBreakpoint (int breakIndex, KrkCodeObject **funcOut, size_t *offsetOut, int *flagsOut, int *enabledOut) |
Retreive information on a breakpoint. More... | |
void | krk_debug_dumpStack (FILE *f, KrkCallFrame *frame) |
Print the elements on the stack. More... | |
void | krk_debug_init (void) |
Initialize debugger state. Call exactly once per VM. | |
Detailed Description
Functions for debugging bytecode execution.
This header provides functions for disassembly bytecode to readable instruction traces, mapping bytecode offsets to source code lines, and handling breakpoint instructions.
Several of these functions are also exported to user code in the dis module.
Note that these functions are not related to manage code exception handling, but instead inteaded to provide a low level interface to the VM's execution process and allow for the implementation of debuggers and debugger extensions.
Definition in file debug.h.
Macro Definition Documentation
◆ KRK_BREAKPOINT_NORMAL
#define KRK_BREAKPOINT_NORMAL 0 |
◆ KRK_BREAKPOINT_ONCE
#define KRK_BREAKPOINT_ONCE 1 |
◆ KRK_BREAKPOINT_REPEAT
#define KRK_BREAKPOINT_REPEAT 2 |
After this breakpoint has fired and execution resumes, the interpreter should re-enable it to fire again until it is removed by a call to krk_debug_removeBreakpoint()
Function Documentation
◆ krk_debug_addBreakpointCodeOffset()
int krk_debug_addBreakpointCodeOffset | ( | KrkCodeObject * | codeObject, |
size_t | offset, | ||
int | flags | ||
) |
Add a breakpoint to the given code object.
A new breakpoint is added to the breakpoint table and the code object's bytecode is overwritten to insert the OP_BREAKPOINT instruction.
Callers must ensure that offset
points to the opcode portion of an instruction, and not an operand, or the attempt to add a breakpoint can corrupt the bytecode.
- Parameters
-
codeObject KrkCodeObject* for the code object to break on. offset Bytecode offset to insert the breakpoint at. flags Allows configuring the disposition of the breakpoint.
- Returns
- A breakpoint identifier handle on success, or -1 on failure.
◆ krk_debug_addBreakpointFileLine()
int krk_debug_addBreakpointFileLine | ( | KrkString * | filename, |
size_t | line, | ||
int | flags | ||
) |
Add a breakpoint to the given line of a file.
The interpreter will scan all code objects and attach a breakpoint instruction to the first such object that has a match filename and contains an instruction with a matching line mapping. Breakpoints can only be added to code which has already been compiled.
- Parameters
-
filename KrkString * representation of a source code filename to look for. line The line to set the breakpoint at. flags Allows configuring the disposition of the breakpoint.
- Returns
- A breakpoint identifier handle on success, or -1 on failure.
◆ krk_debug_disableBreakpoint()
int krk_debug_disableBreakpoint | ( | int | breakpointId | ) |
Disable a breakpoint.
Restores the bytecode instructions for the given breakpoint if it is currently enabled.
No error is returned if the breakpoint was already disabled.
- Parameters
-
breakpointId The breakpoint to disable.
- Returns
- 0 on success, 1 if the breakpoint identifier is invalid.
◆ krk_debug_dumpStack()
void krk_debug_dumpStack | ( | FILE * | file, |
KrkCallFrame * | frame | ||
) |
Print the elements on the stack.
Prints the elements on the stack for the current thread to file
, highlighting frame
as the activate call point and indicating where its arguments start.
When tracing is enabled, we will present the elements on the stack with a safe printer; the format of values printed by krk_printValueSafe will look different from those printed by printValue, but they guarantee that the VM will never be called to produce a string, which would result in a nasty infinite recursion if we did it while trying to trace the VM!
◆ krk_debug_dumpTraceback()
void krk_debug_dumpTraceback | ( | void | ) |
Safely dump a traceback to stderr.
Wraps krk_dumpTraceback() so it can be safely called from a debugger.
Safely dump a traceback to stderr.
Since traceback printing may involve calling into user code, we clear the debugging bits. Then we make a new exception to attach a traceback to.
◆ krk_debug_enableBreakpoint()
int krk_debug_enableBreakpoint | ( | int | breakpointId | ) |
◆ krk_debug_examineBreakpoint()
int krk_debug_examineBreakpoint | ( | int | breakIndex, |
KrkCodeObject ** | funcOut, | ||
size_t * | offsetOut, | ||
int * | flagsOut, | ||
int * | enabledOut | ||
) |
Retreive information on a breakpoint.
Can be called by debuggers to examine the details of a breakpoint by its handle. Information is returned through the pointers provided as parameters.
- Parameters
-
breakIndex Breakpoint handle to examine. funcOut (Out) The code object this breakpoint is in. offsetOut (Out) The bytecode offset within the code object where the breakpoint is located. flagsOut (Out) The configuration flags for the breakpoint. enabledOut (Out) Whether the breakpoint is enabled or not.
- Returns
- 0 on success, -1 on out of range, -2 if the selected slot was removed.
◆ krk_debug_registerCallback()
int krk_debug_registerCallback | ( | KrkDebugCallback | hook | ) |
Register a debugger callback.
The registered function hook
will be called when an OP_BREAKPOINT instruction is encountered. The debugger is provided a pointer to the active frame and can use functions from the krk_debug_* suite to examine the thread state, execute more code, and resume execution.
The debugger hook will be called from the thread that encountered the breakpoint, and should return one of the KRK_DEBUGGER_ status values to inform the VM of what action to take.
- Parameters
-
hook The hook function to attach.
- Returns
- 0 if the hook was registered; 1 if a hook was already registered, in which case the new hook has not been registered.
◆ krk_debug_removeBreakpoint()
int krk_debug_removeBreakpoint | ( | int | breakpointId | ) |
Remove a breakpoint from the breakpoint table.
Removes the breakpoint breakpointId
from the breakpoint table, restoring the bytecode instruction for it if it was enabled.
- Parameters
-
breakpointId The breakpoint to remove.
- Returns
- 0 on success, 1 if the breakpoint identifier is invalid.
◆ krk_debugBreakpointHandler()
int krk_debugBreakpointHandler | ( | void | ) |
◆ krk_debuggerHook()
int krk_debuggerHook | ( | KrkCallFrame * | frame | ) |
◆ krk_disassembleCodeObject()
void krk_disassembleCodeObject | ( | FILE * | f, |
KrkCodeObject * | func, | ||
const char * | name | ||
) |
Print a disassembly of 'func' to the stream 'f'.
Generates and prints a bytecode disassembly of the code object 'func', writing it to the requested stream.
- Parameters
-
f Stream to write to. func Code object to disassemble. name Function name to display in disassembly output.
◆ krk_disassembleInstruction()
size_t krk_disassembleInstruction | ( | FILE * | f, |
KrkCodeObject * | func, | ||
size_t | offset | ||
) |
Print a disassembly of a single opcode instruction.
Generates and prints a bytecode disassembly for one instruction from the code object 'func' at byte offset 'offset', printing the result to the requested stream and returning the size of the instruction.
- Parameters
-
f Stream to write to. func Code object to disassemble. offset Byte offset of the instruction to disassemble.
- Returns
- The size of the instruction in bytes.