value.h File Reference
Definitions for primitive stack references. More...
Include dependency graph for value.h:
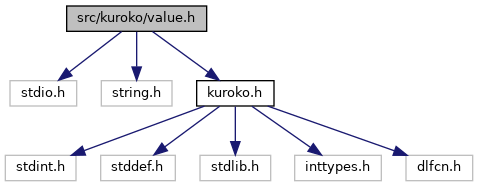
This graph shows which files directly or indirectly include this file:

Go to the source code of this file.
Data Structures | |
struct | KrkValueArray |
Flexible vector of stack references. More... | |
union | KrkValueDbl |
Macros | |
#define | _krk_valuesSame(a, b) (a == b) |
#define | KRK_VAL_MASK_BOOLEAN ((uint64_t)0xFFFC000000000000) /* 1..1100 */ |
#define | KRK_VAL_MASK_INTEGER ((uint64_t)0xFFFD000000000000) /* 1..1101 */ |
#define | KRK_VAL_MASK_HANDLER ((uint64_t)0xFFFE000000000000) /* 1..1110 */ |
#define | KRK_VAL_MASK_NONE ((uint64_t)0xFFFF000000000000) /* 1..1111 */ |
#define | KRK_VAL_MASK_KWARGS ((uint64_t)0x7FFC000000000000) /* 0..1100 */ |
#define | KRK_VAL_MASK_OBJECT ((uint64_t)0x7FFD000000000000) /* 0..1101 */ |
#define | KRK_VAL_MASK_NOTIMPL ((uint64_t)0x7FFE000000000000) /* 0..1110 */ |
#define | KRK_VAL_MASK_NAN ((uint64_t)0x7FFC000000000000) |
#define | KRK_VAL_MASK_LOW ((uint64_t)0x0000FFFFFFFFFFFF) |
#define | _krk_sanitize(ptr) (ptr) |
#define | KRK_HEAP_TAG 0 |
#define | NONE_VAL() ((KrkValue)(KRK_VAL_MASK_LOW | KRK_VAL_MASK_NONE)) |
#define | NOTIMPL_VAL() ((KrkValue)(KRK_VAL_MASK_LOW | KRK_VAL_MASK_NOTIMPL)) |
#define | BOOLEAN_VAL(value) ((KrkValue)(((uint64_t)(value) & KRK_VAL_MASK_LOW) | KRK_VAL_MASK_BOOLEAN)) |
#define | INTEGER_VAL(value) ((KrkValue)(((uint64_t)(value) & KRK_VAL_MASK_LOW) | KRK_VAL_MASK_INTEGER)) |
#define | KWARGS_VAL(value) ((KrkValue)((uint32_t)(value) | KRK_VAL_MASK_KWARGS)) |
#define | OBJECT_VAL(value) ((KrkValue)((_krk_sanitize((uintptr_t)(value)) & KRK_VAL_MASK_LOW) | KRK_VAL_MASK_OBJECT)) |
#define | HANDLER_VAL(ty, ta) ((KrkValue)((uint64_t)((((uint64_t)ty) << 32) | ((uint32_t)ta)) | KRK_VAL_MASK_HANDLER)) |
#define | FLOATING_VAL(value) (((KrkValueDbl){.dbl = (value)}).val) |
#define | KRK_VAL_TYPE(value) ((value) >> 48) |
#define | KRK_IX(value) ((uint64_t)((value) & KRK_VAL_MASK_LOW)) |
#define | KRK_SX(value) ((uint64_t)((value) & 0x800000000000)) |
#define | AS_INTEGER(value) ((krk_integer_type)(KRK_SX(value) ? (KRK_IX(value) | KRK_VAL_MASK_NONE) : (KRK_IX(value)))) |
#define | AS_BOOLEAN(value) AS_INTEGER(value) |
#define | AS_NOTIMPL(value) ((krk_integer_type)((value) & KRK_VAL_MASK_LOW)) |
#define | AS_HANDLER(value) ((uint64_t)((value) & KRK_VAL_MASK_LOW)) |
#define | AS_OBJECT(value) ((KrkObj*)(uintptr_t)(((value) & KRK_VAL_MASK_LOW) | KRK_HEAP_TAG)) |
#define | AS_FLOATING(value) (((KrkValueDbl){.val = (value)}).dbl) |
#define | IS_INTEGER(value) ((((value) >> 48L) & (KRK_VAL_MASK_HANDLER >> 48L)) == (KRK_VAL_MASK_BOOLEAN >> 48L)) |
#define | IS_BOOLEAN(value) (((value) >> 48L) == (KRK_VAL_MASK_BOOLEAN >> 48L)) |
#define | IS_NONE(value) (((value) >> 48L) == (KRK_VAL_MASK_NONE >> 48L)) |
#define | IS_HANDLER(value) (((value) >> 48L) == (KRK_VAL_MASK_HANDLER >> 48L)) |
#define | IS_OBJECT(value) (((value) >> 48L) == (KRK_VAL_MASK_OBJECT >> 48L)) |
#define | IS_KWARGS(value) (((value) >> 48L) == (KRK_VAL_MASK_KWARGS >> 48L)) |
#define | IS_NOTIMPL(value) (((value) >> 48L) == (KRK_VAL_MASK_NOTIMPL >> 48L)) |
#define | IS_FLOATING(value) (((value) & KRK_VAL_MASK_NAN) != KRK_VAL_MASK_NAN) |
#define | AS_HANDLER_TYPE(value) (AS_HANDLER(value) >> 32) |
#define | AS_HANDLER_TARGET(value) (AS_HANDLER(value) & 0xFFFFFFFF) |
#define | IS_HANDLER_TYPE(value, type) (IS_HANDLER(value) && AS_HANDLER_TYPE(value) == type) |
#define | KWARGS_SINGLE (INT32_MAX) |
#define | KWARGS_LIST (INT32_MAX-1) |
#define | KWARGS_DICT (INT32_MAX-2) |
#define | KWARGS_NIL (INT32_MAX-3) |
#define | KWARGS_UNSET (0) |
#define | PRIkrk_int "%" PRId64 |
#define | PRIkrk_hex "%" PRIx64 |
Typedefs | |
typedef uint64_t | KrkValue |
Enumerations | |
enum | KrkValueType { KRK_VAL_BOOLEAN = 0xFFFC , KRK_VAL_INTEGER = 0xFFFD , KRK_VAL_HANDLER = 0xFFFE , KRK_VAL_NONE = 0xFFFF , KRK_VAL_KWARGS = 0x7FFC , KRK_VAL_OBJECT = 0x7FFD , KRK_VAL_NOTIMPL = 0x7FFE } |
Tag enum for basic value types. More... | |
Functions | |
KrkValue | krk_parse_int (const char *start, size_t width, unsigned int base) |
KrkValue | krk_parse_float (const char *start, size_t width) |
Parse a string into a float. More... | |
Detailed Description
Definitions for primitive stack references.
Definition in file value.h.
Macro Definition Documentation
◆ KRK_HEAP_TAG
#define KRK_HEAP_TAG 0 |
Enumeration Type Documentation
◆ KrkValueType
enum KrkValueType |
Function Documentation
◆ krk_parse_float()
KrkValue krk_parse_float | ( | const char * | s, |
size_t | l | ||
) |
Parse a string into a float.
The approach we take here is to collect all of the digits left of the exponent (if present), convert them to a big int disregarding the radix point, then multiply or divide that by an appropriate power of ten based on the exponent and location of the radix point. The division step uses are long.__truediv__
to get accurate conversions of fractions to floats.
May raise exceptions if parsing fails, either here or in integer parsing.
- Parameters
-
s String to parse. l Length of string to parse.
- Returns
- A Kuroko float value, or None on exceptin.
Definition at line 2820 of file obj_long.c.