scanner.h File Reference
Definitions used by the token scanner. More...
This graph shows which files directly or indirectly include this file:
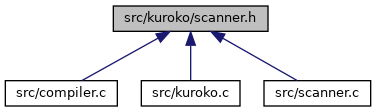
Go to the source code of this file.
Data Structures | |
struct | KrkToken |
A token from the scanner. More... | |
struct | KrkScanner |
Token scanner state. More... | |
Functions | |
KrkScanner | krk_initScanner (const char *src) |
Initialize the compiler to scan tokens from 'src'. | |
KrkToken | krk_scanToken (KrkScanner *) |
Read the next token from the scanner. | |
void | krk_ungetToken (KrkScanner *, KrkToken token) |
Push a token back to the scanner to be reprocessed. More... | |
void | krk_rewindScanner (KrkScanner *, KrkScanner to) |
Rewind the scanner to a previous state. More... | |
KrkScanner | krk_tellScanner (KrkScanner *) |
Retreive a copy of the current scanner state. More... | |
Detailed Description
Definitions used by the token scanner.
Definition in file scanner.h.
Function Documentation
◆ krk_rewindScanner()
void krk_rewindScanner | ( | KrkScanner * | scanner, |
KrkScanner | to | ||
) |
◆ krk_tellScanner()
KrkScanner krk_tellScanner | ( | KrkScanner * | scanner | ) |
Retreive a copy of the current scanner state.
Used with krk_rewindScanner() to implement rescanning for comprehensions.
◆ krk_ungetToken()
void krk_ungetToken | ( | KrkScanner * | scanner, |
KrkToken | token | ||
) |